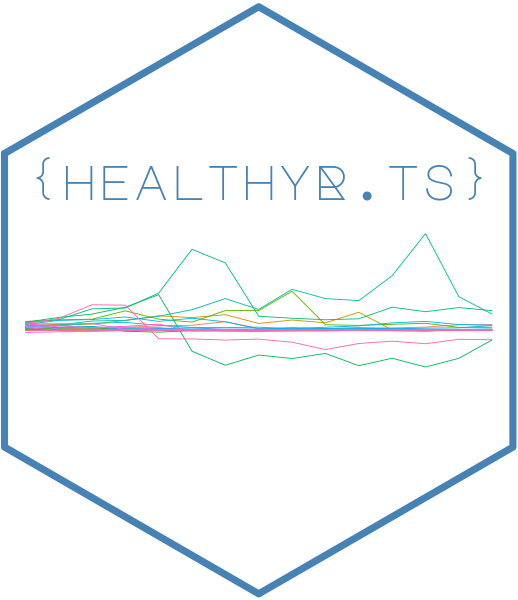
Geometric Brownian Motion
Source:R/ts-geometric-brownian-motion-augment.R
ts_geometric_brownian_motion_augment.Rd
Create a Geometric Brownian Motion.
Usage
ts_geometric_brownian_motion_augment(
.data,
.date_col,
.value_col,
.num_sims = 10,
.time = 25,
.mean = 0,
.sigma = 0.1,
.delta_time = 1/365
)
Arguments
- .data
The data you are going to pass to the function to augment.
- .date_col
The column that holds the date
- .value_col
The column that holds the value
- .num_sims
Total number of simulations.
- .time
Total time of the simulation.
- .mean
Expected return
- .sigma
Volatility
- .delta_time
Time step size.
Details
Geometric Brownian Motion (GBM) is a statistical method for modeling the evolution of a given financial asset over time. It is a type of stochastic process, which means that it is a system that undergoes random changes over time.
GBM is widely used in the field of finance to model the behavior of stock prices, foreign exchange rates, and other financial assets. It is based on the assumption that the asset's price follows a random walk, meaning that it is influenced by a number of unpredictable factors such as market trends, news events, and investor sentiment.
The equation for GBM is:
where S is the price of the asset, t is time, m is the expected return on the asset, s is the volatility of the asset, and dW is a small random change in the asset's price.
GBM can be used to estimate the likelihood of different outcomes for a given asset, and it is often used in conjunction with other statistical methods to make more accurate predictions about the future performance of an asset.
This function provides the ability of simulating and estimating the parameters of a GBM process. It can be used to analyze the behavior of financial assets and to make informed investment decisions.
See also
Other Data Generator:
tidy_fft()
,
ts_brownian_motion_augment()
,
ts_brownian_motion()
,
ts_geometric_brownian_motion()
,
ts_random_walk()
Examples
rn <- rnorm(31)
df <- data.frame(
date_col = seq.Date(from = as.Date("2022-01-01"),
to = as.Date("2022-01-31"),
by = "day"),
value = rn
)
ts_geometric_brownian_motion_augment(
.data = df,
.date_col = date_col,
.value_col = value
)
#> # A tibble: 291 × 3
#> sim_number date_col value
#> <fct> <date> <dbl>
#> 1 actual_data 2022-01-01 -1.46
#> 2 actual_data 2022-01-02 -0.335
#> 3 actual_data 2022-01-03 -1.89
#> 4 actual_data 2022-01-04 1.10
#> 5 actual_data 2022-01-05 -0.950
#> 6 actual_data 2022-01-06 0.595
#> 7 actual_data 2022-01-07 -0.299
#> 8 actual_data 2022-01-08 -0.0139
#> 9 actual_data 2022-01-09 0.415
#> 10 actual_data 2022-01-10 2.75
#> # ℹ 281 more rows