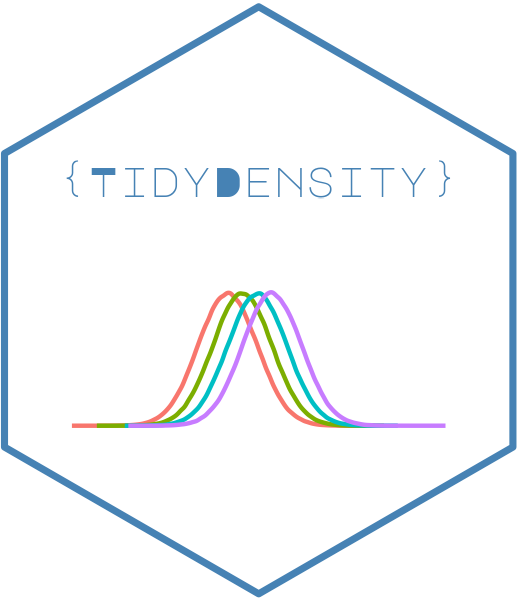
Perform quantile normalization on a numeric matrix/data.frame
Source:R/utils-quantile-normalize.R
quantile_normalize.Rd
This function will perform quantile normalization on two or more distributions of equal length. Quantile normalization is a technique used to make the distribution of values across different samples more similar. It ensures that the distributions of values for each sample have the same quantiles. This function takes a numeric matrix as input and returns a quantile-normalized matrix.
Arguments
- .data
A numeric matrix where each column represents a sample.
- .return_tibble
A logical value that determines if the output should be a tibble. Default is 'FALSE'.
Value
A list object that has the following:
A numeric matrix that has been quantile normalized.
The row means of the quantile normalized matrix.
The sorted data
The ranked indices
Details
This function performs quantile normalization on a numeric matrix by following these steps:
Sort each column of the input matrix.
Calculate the mean of each row across the sorted columns.
Replace each column's sorted values with the row means.
Unsort the columns to their original order.
See also
rowMeans
: Calculate row means.
apply
: Apply a function over the margins of an array.
order
: Order the elements of a vector.
Other Utility:
check_duplicate_rows()
,
convert_to_ts()
,
tidy_mcmc_sampling()
,
util_beta_aic()
,
util_binomial_aic()
,
util_cauchy_aic()
,
util_chisq_aic()
,
util_exponential_aic()
,
util_f_aic()
,
util_gamma_aic()
,
util_generalized_beta_aic()
,
util_generalized_pareto_aic()
,
util_geometric_aic()
,
util_hypergeometric_aic()
,
util_inverse_burr_aic()
,
util_inverse_pareto_aic()
,
util_inverse_weibull_aic()
,
util_logistic_aic()
,
util_lognormal_aic()
,
util_negative_binomial_aic()
,
util_normal_aic()
,
util_paralogistic_aic()
,
util_pareto1_aic()
,
util_pareto_aic()
,
util_poisson_aic()
,
util_t_aic()
,
util_triangular_aic()
,
util_uniform_aic()
,
util_weibull_aic()
,
util_zero_truncated_binomial_aic()
,
util_zero_truncated_geometric_aic()
,
util_zero_truncated_negative_binomial_aic()
,
util_zero_truncated_poisson_aic()
Examples
# Create a sample numeric matrix
data <- matrix(rnorm(20), ncol = 4)
# Perform quantile normalization
normalized_data <- quantile_normalize(data)
#> Warning: There are duplicated ranks the input data.
normalized_data
#> $normalized_data
#> [,1] [,2] [,3] [,4]
#> [1,] -0.3544741 -1.1887651 0.1402623 0.1402623
#> [2,] -1.1887651 0.1402623 -0.3544741 -0.3544741
#> [3,] 0.1402623 -0.3544741 0.6082141 -1.1887651
#> [4,] 1.5541985 1.5541985 -1.1887651 0.6082141
#> [5,] 0.6082141 0.6082141 1.5541985 1.5541985
#>
#> $row_means
#> [1] -1.1887651 -0.3544741 0.1402623 0.6082141 1.5541985
#>
#> $duplicated_ranks
#> [,1] [,2] [,3] [,4]
#> [1,] 5 2 1 2
#> [2,] 4 1 5 4
#> [3,] 2 3 3 1
#> [4,] 3 4 4 5
#>
#> $duplicated_rank_row_indices
#> [1] 1 3 4 5
#>
#> $duplicated_rank_data
#> [,1] [,2] [,3] [,4]
#> [1,] -0.006202427 -0.1106733 -1.2344467 0.8044256
#> [2,] 2.531894845 0.1679075 0.7490085 -0.7630899
#> [3,] 0.212224663 0.6147875 1.2798579 -0.0333282
#> [4,] -2.150115206 -0.1142912 0.4928258 1.7902539
#>
as.data.frame(normalized_data$normalized_data) |>
sapply(function(x) quantile(x, probs = seq(0, 1, 1 / 4)))
#> V1 V2 V3 V4
#> 0% -1.1887651 -1.1887651 -1.1887651 -1.1887651
#> 25% -0.3544741 -0.3544741 -0.3544741 -0.3544741
#> 50% 0.1402623 0.1402623 0.1402623 0.1402623
#> 75% 0.6082141 0.6082141 0.6082141 0.6082141
#> 100% 1.5541985 1.5541985 1.5541985 1.5541985
quantile_normalize(
data.frame(rnorm(30),
rnorm(30)),
.return_tibble = TRUE)
#> Warning: There are duplicated ranks the input data.
#> $normalized_data
#> # A tibble: 30 × 2
#> rnorm.30. rnorm.30..1
#> <dbl> <dbl>
#> 1 -0.341 1.51
#> 2 -0.744 0.983
#> 3 0.109 0.645
#> 4 0.346 -0.150
#> 5 1.26 -0.316
#> 6 -0.551 -0.874
#> 7 0.346 -1.60
#> 8 0.719 -0.0315
#> 9 -1.81 -0.670
#> 10 -1.37 -0.403
#> # ℹ 20 more rows
#>
#> $row_means
#> # A tibble: 30 × 1
#> value
#> <dbl>
#> 1 -1.81
#> 2 -1.60
#> 3 -1.37
#> 4 -1.18
#> 5 -1.01
#> 6 -0.874
#> 7 -0.744
#> 8 -0.670
#> 9 -0.551
#> 10 -0.403
#> # ℹ 20 more rows
#>
#> $duplicated_ranks
#> # A tibble: 2 × 1
#> value
#> <int>
#> 1 8
#> 2 8
#>
#> $duplicated_rank_row_indices
#> # A tibble: 1 × 1
#> row_index
#> <int>
#> 1 23
#>
#> $duplicated_rank_data
#> # A tibble: 2 × 1
#> value
#> <dbl>
#> 1 0.727
#> 2 -0.566
#>